What are Parameters And What Is Their Use
PARAMETERS help the programmer write a PROCEDURE that provides the flexibility to draw shapes of different sizes, colors, outline width, etc. using just one single procedure.
If the LOGO language did not have the feature of PARAMETERS, we would have to write a new program every time we needed to draw the same shape with a different size, different outline thickness, and color.
Parameters are a slightly advanced concept for very young learners. So, this chapter and all the following chapters in the LOGO section can be skipped on initial learning. We will explain the Parameters concept by using an example. Although parameters apply to any shape, we will use SQUARE as an example to describe the Parameters concept.
Extending the “TO” Command By Including Parameters
On the LOGO Procedures page, we learned how to draw a SQUARE using the “TO” command. The table below shows two ways to draw a square (or any shape).
Using the technique from LOGO PROCEDURES page
LOGO Procedures technique is described in the middle column of the table shaded green. From this table, we see that we have to write a new program every time we like to draw a square of a different size. This is indicated by numbers colored in red (100, 150, and 200). Writing a new program to draw a shape of a different size is very inefficient and inflexible. What if you want to draw a SQUARE of any size? It would be really neat if we were able to draw a square of any size using just one program. Parameters give you the flexibility to achieve this objective.
Using Parameters
Alternatively, we can use Parameters. Using parameters to draw a shape is shown in the third column of the Table (shaded light blue). This column shows how we can have the turtle draw a square (or any other shape) of any size using Parameters. The concept of Parameters is explained in more detail below.
How Parameters Work
Below we show how to use parameters to draw a shape of any size.
We learned about the TO command to draw a square on the LOGO Procedures page. To make use of parameters, we extend the TO command by adding a parameter. The statement below shows how to add a parameter ‘length’ to the TO command.
TO SQUARE :length
‘length’ is an example of a parameter. The parameter ‘length’ is a variable, meaning it can be assigned a different value every time the TO procedure is called by your main program. Thus, Parameters allow us to pass a new value to the PROCEDURE.
Notice that the parameter in the TO statement starts with a colon (:).
How to Use Parameters
The third column in the table shaded light blue shows how parameters are used to draw SQUARES with sides of different lengths using the statement TO SQUARE :length.
Using the parameter approach, the procedure is written only once. The procedure statement includes the parameter :length. as in statement TO SQUARE :length.
When the procedure TO SQUARE :length is called by the calling program, the calling program passes the value of the length parameter. The passing of the value of the parameter is done by the calling program by a one-line statement such as SQUARE 150. This statement will draw a square with sides = 150 pixels.
If the calling program calls the procedure by a statement SQUARE 200, the program will draw a square of side length = 200 pixels.
These statements call the TO procedure and pass the parameter “length” 100, 150, or 200 as in the example in the third column of the table.
Note that the parameters must be defined at the top of the LOGO program. You will see it in the example below.
Using Parameters vs. Not Using Parameters

More information on Parameters is at this site.
https://cs.brown.edu/courses/bridge/1997/Resources/LogoTutorial.html
Draw Square of Any Size Using Parameters
The program below uses parameter to draw ten squares of different sizes. It uses just one program, instead of ten programs that would be needed if we did not use parameters.
PROGRAM EXAMPLE: PARAMETER USAGE IN LOGO
TO SQUARE :LENGTH ;1) Define length as parameter that can be assigned different values
REPEAT 4 [FORWARD :LENGTH RIGHT 90 ] :2)
END
REPEAT 10 [SQUARE REPCOUNT * 10] ;3) Call SQUARE Procedure
Detailed Explanation of the Program
Let us first look at the TO procedure that defines the procedure to draw a square. (Code line 1)
Instead of using REPEAT 4[FORWARD 200 RIGHT 90] statement, the procedure uses REPEAT 4[FORWARD :LENGTH RIGHT 90] statement (Code line 2).
The last line 3) in the above program is the calling (main) program. It makes a call to the TO SQUARE :LENGTH procedure and replaces the LENGTH parameter with REPCOUNT*10.
Let us look deeper into how the program draws many squares using a single statement. On the first iteration of the REPEAT 10 [SQUARE REPCOUNT * 10] command, the program will draw a square of size 10. Remember REPCOUNT is always 1 on the first iteration of the REPEAT loop, so REPCOUNT * 10 = 10.
The second repeat iteration, REPCOUNT = 2 making REPCOUNT * 10 = 20. Therefore, REPEAT 10 [SQUARE REPCOUNT * 10] will draw a square of length = 20.
On the last repeat count of 10, REPCOUNT = 10, so the size of the square is 10*10 = 100. Thus, the program will draw ten squares of side length from 10, 20, 30, …. to 100. Below is the output of the program.
Below is the output of the program.

Now, we will make it more interesting by using two parameters.
Use Two Parameters – Draw Polygon of Any Number of Sides
Now we know how to draw a square using parameters. But what if we want to draw a polygon? Since polygons can have any number of sides from 3, 4, 5, or any larger number, it will make sense to define the number of ”sides” as one parameter. Let’s use “length” as the second parameter (same as in the previous program).
If we want to draw a triangle, the calling program will pass the parameter sides = 3. In case we want to draw a square, the calling program will pass parameter sides = 4. For the case of an octagon, the calling program will pass the parameter sides = 8, and so on.
The program uses the second parameter “length” to choose the length of the polygon’s sides just as we did in the program to draw squares of any side length.
Before we start to draw polygons with any number of sides, the Table that we discussed on the Basic Geometry Concepts page is reproduced below for reference. This Table lists the exterior angles for polygons of the different number of sides.
Polygon’s Interior and Exterior Angles
POLYGON SHAPE | NUMBER OF SIDES | EXTERIOR ANGLE | INTERIOR ANGLE |
---|---|---|---|
Triangle | 3 | 360 ÷ 3 = 120° | 60° |
Square | 4 | 360 ÷ 4 = 90° | 90° |
Pentagon | 5 | 360 ÷ 5 = 72° | 108° |
Hexagon | 6 | 360 ÷ 6 = 60° | 120° |
Octagon | 8 | 360 ÷ 8 = 45° | 135° |
Decagon | 10 | 360 ÷ 10 = 36° | 144° |
“TO” Procedure With Two Parameters (Number of Sides and Length)
The calling program passes two parameters “sides” and “length” to the called procedure.
The program below uses two parameters :sides and :length. The calling program passes the sides parameter = 5 to draw a pentagon. The Table above shows that the external angle of a pentagon = 360/5 = 72 degrees. Therefore, the TO procedure asks the turtle turn right by an angle 360/sides = 360/5 = 72 degrees.
PROGRAM EXAMPLE: POLYGON USING TWO PARAMETERS
TO POLYGON :sides :length ;1) Define sides and length as parameters.
REPEAT :sides [FORWARD :length RIGHT 360/:sides] ;2)
END
; Main program below.
POLYGON 5 200 ; 3) Call POLYGON procedure to draw
;a pentagon of side 200. The angle is 360/5 = 72.
Detailed Explanation of the Program:
Code line 1)
TO POLYGON :sides :length
defines a Procedure with two parameters sides and length.
Code line 2) is the main body of the TO procedure.
REPEAT :sides [FORWARD :length RIGHT 360/:sides]
When the main program calls the TO procedure using the statement POLYGON 5 200, it passes the parameter sides = 5 and length = 200.
The image produced by the program is show below.

To draw a square, we call the procedure as below:
POLYGON 4 50 ; this will draw a square of side 50
To draw a triangle, we call the procedure as below:
POLYGON 3, 100 ;This will draw a triangle of side 100.
In summary, Procedures with parameters take “inputs” so that the information they use is different each time the procedure is called.
In the following, we will use the TO procedure with three parameters.
Three Parameters: sides, length, and color
You can use any number of parameters in defining your TO procedure. The previous program used “sides” and “length” as the parameters. If we want to draw a shape with any color, we can specify a third parameter “color” as in the example below.
PROGRAM EXAMPLE: DRAW POLYGON WITH THREE PARAMETERS
TO POLYGON :SIDES :LENGTH :COLOR ;1)
SETPENSIZE 3 ;2)
REPEAT :SIDES[FORWARD :LENGTH RIGHT 360/:SIDES] ;3)
HIDETURTLE ;4)
End
; MAIN PROGRAM BELOW.
POLYGON 8 40 12 ;5)
Detailed Explanation of the Program:
In the program above,
Line 1) TO POLYGON :SIDES :LENGTH :COLOR
The TO command defines a procedure called “POLYGON” and uses three parameters “SIDES”, “LENGTH”, and “COLOR”.
Lines 2) SETPENSIZE 3
The above code sets the pen size to ‘3’ to make the pen draw thicker outlines of the polygon.
Line 3) REPEAT :SIDES[FORWARD :LENGTH RIGHT 360/:SIDES]
This Line defines the functionality of the procedure “POLYGON”.
Line 4) HIDETURTLE
In line 4, we use the HIDETURTLE command to hide the turtle so that it does not show on the final drawn picture of the polygon.
The Main Program
Line 5) POLYGON 8 40 12
Line 5) is the main program. The main program calls the POLYGON procedure with the statement POLYGON 8 40 12.
Here, ‘8’, ’40’, and ’12’ are the parameters passed by the main program to the Procedure POLYGON.
The number “8” specifies the parameter SIDES corresponding to the number of sides of a pentagon. The “8” in the main program statement POLYGON 8 40 12 replaces the parameter :SIDES in the Procedure statement TO POLYGON :SIDES :LENGTH :COLOR. This statement will make the turtle draw a pentagon.
Similarly, the number “40 in statement POLYGON 8 40 12 replaces the parameter :LENGTH in Procedure statement TO POLYGON :SIDES :LENGTH :COLOR. This statement will make the turtle draw a pentagon of side length 40.
The number “12” in statement POLYGON 8 40 12 replaces the parameter :COLOR in Procedure statement TO POLYGON :SIDES :LENGTH :COLOR. Number “12” selects the color of the outline of the octagon. The number “12” corresponds to tan color (see color index table on the Pen Control and Color Control page.
Thus, the turtle will draw an octagon with thicker outlines in tan color. Below is the output of the above program.
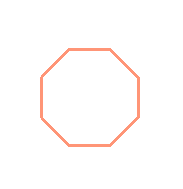
Draw a Simple House Using Parameters
How about we draw a simple house using the parameters that we used in the previous examples? There are no new concepts in this program; it just uses all the concepts we have learned so far such as Pen Control and Color Control and parameters.
PROGRAM EXAMPLE: DRAW A HOUSE USING PARAMETERS
TO POLYGON :SIDES :LENGTH
REPEAT :SIDES[FD :LENGTH RIGHT 360/:SIDES]
END
TO HOUSE :LENGTH
; Call procedure POLYGON to draw a square for the
; front of the house.
POLYGON 4 :LENGTH
;
FORWARD :LENGTH ; Move turtle to top left
; of the house.
RIGHT 30 ; Turn turtle right 30 degrees to
; get ready to draw the roof.
; Call procedure POLYGON to draw triangular roof.
POLYGON 3 :LENGTH
;
RIGHT 150 ;Turn turtle right 150 degrees to face down.
FORWARD :LENGTH ; Move turtle to the
;left bottom of the house.
LEFT 90 ; Turn left to get ready to draw the door.
FORWARD :LENGTH/4
LEFT 90
; Call procedure POLYGON to draw the door.
POLYGON 4 :LENGTH/2
END
; Main Program below
CLEARSCREEN
PENDOWN
SETPENSIZE 3
SETPENCOLOR 4
HOUSE 160; Call the procedure HOUSE
Below is the program output.

Draw a House With Color Fill
This house looks nice. But it will look nicer if fill the house door and roof with colors. Refer to the color index table and FILL command from LOGO Pen Control and Color Control page. Below is the program that fills some color into the house.
PROGRAM EXAMPLE: DRAW A HOUSE WITH COLOR FILL
TO POLYGON :SIDES :LENGTH
REPEAT :SIDES[FD :LENGTH RIGHT 360/:SIDES]
END
TO HOUSE :LENGTH
; Call procedure POLYGON to draw a square for the front of the house.
POLYGON 4 :LENGTH
;
FORWARD :LENGTH ; Move turtle to top left of the house.
RIGHT 30 ; Turn turtle right 30 degrees to get ready to draw the roof.
; Call procedure POLYGON to draw triangular roof.
POLYGON 3 :LENGTH
;
RT 30
PU
FD 45
SETFLOODCOLOR 6
Fill
BK 45
LT 30
PD
;
RIGHT 150 ; Turn turtle right 150 degrees to face down.
FORWARD :LENGTH ; Move turtle to the left bottom of the house.
LEFT 90 ; Turn left to get ready to draw the door.
FORWARD :LENGTH/4
LEFT 90
; Call procedure POLYGON to draw the door.
SETPENCOLOR 11 ;Set door color.
POLYGON 4 :LENGTH/2
;
RT 45
PU
FD 10
SETFLOODCOLOR 14
Fill
;
END
; Main Program below
CLEARSCREEN
SETPENSIZE 3
SETPENCOLOR 4
HOUSE 160; Call the procedure HOUSE
And here is the image of our new house.

Draw a Rocket Using Parameters
In this section, we will use TO command with parameters to draw a rocket shape.
PROGRAM EXAMPLE: DRAW A ROCKET SHAPE
TO BODY :HEIGHT :WIDTH ;1)
REPEAT 2[FORWARD :HEIGHT RIGHT 90 FORWARD :WIDTH RIGHT 90]
END
TO NOSECONE :SIDES :LENGTH ;2)
REPEAT :SIDES [FORWARD :LENGTH RIGHT 360/:SIDES]
END
TO ROCKET ;3)
PENDOWN
BODY 80 40; Parameter HEIGHT = 40 and Parameter WIDTH = 40
FORWARD 80
RIGHT 30
NOSECONE 3 40; Parameter SIDES = 3 and parameter LENGTH = 40
PENUP
LEFT 30
BACK 80
RIGHT 45
BACK 40
PENDOWN
REPEAT 2[Fd 40 rt 90 fd 30 rt 90]; Draw a rectangle with sides 40 and 30 for exhaust pipe.
PENUP
FORWARD 40
RIGHT 45
FORWARD 40
RIGHT 45
PENDOWN
REPEAT 2[Fd 40 rt 90 fd 30 rt 90]; ; Draw a rectangle with sides 40 and 30 for exhaust pipe.
LEFT 135
; Fill BODY with green color
PENUP
FORWARD 20
LEFT 90
FORWARD 20
SETFLOODCOLOR 2; Color index 2 means green color.
FILL
; Fill NOSECONE with yellow color.
RIGHT 90
FORWARD 70
SETFLOODCOLOR 6; Color index 6 means yellow color.
FILL
; Fill Left Exhaust pipe with red color.
BACK 105
LEFT 90
FORWARD 20
SETFLOODCOLOR 4; Color index 4 means red color.
FILL
; Fill Right Exhaust pipe with red color.
BACK 45
SETFLOODCOLOR 4
FILL
HIDETURTLE
END
;Main program
ROCKET; Call procedure ROCKET ;4)
Below is the program’s output.

Detailed Explanation of the Program:
The numbers below correspond to comments in the program above.
1): The procedure BODY draws the main body of the rocket, which is a rectangle colored green in the image. This procedure uses two parameters :HEIGHT (80 in this program) and :WIDTH (40 in this program).
2): The procedure NOSECONE is a polygon procedure using two parameters :LENGTH (40 in this program) and :SIDES (3 in this program). This procedure draws an equilateral triangle of side length = 40.
3): The procedure ROCKET calls the procedures BODY and NOSECONE to build the rocket shape.
The rest of the code moves the turtle around to make the two exhaust outlets of the rocket and fill the colors in the different portions of the rocket.
4): The last line of the program is the main program that call the procedure ROCKET.
Copyright © 2019 – 2024 softwareprogramming4kids.com
All Rights Reserved