Additional Commands in LOGO Language
LOGO has several additional commands. Some of these are the RANDOM command, the PICK command, and commands to hide or unhide the turtle, positioning the turtle at specific coordinates on the screen, and many more.
These commands can be used in creating interesting LOGO programs.
Let us start with how we can use random numbers in LOGO programs.
Random Numbers
When we use an instruction FORWARD 100, the turtle moves forward 100 pixels.
What if we do not want to move the turtle by a fixed number of pixels? LOGO provides the RANDOM command to generate a random number.
RANDOM Command
The RANDOM command has one argument. This command generates a random integer value between 0 and the value of its argument. Suppose you want to move the turtle forward by a random number of pixels between 0 and 99. To do that, you will type the following command:
FORWARD RANDOM 100
Note ‘100’ is the argument in this statement. RANDOM statement will always move the turtle one less than the argument specified.
This command will move the turtle forward by a random number of pixels between 0 and 99. If you run this command again, it will move the turtle forward by a different random number between 0 and 99.
As another example, FORWARD RANDOM 10
will move the turtle forward by a randomly selected number between 0 and 9.
How about turning the turtle by a random angle? If you want to turn the turtle right by a random angle between 0 and 90 degrees, you will type the following command:
RIGHT RANDOM 90
The turtle will turn right by a random number between 0 and 89 degrees.
Run the command below on your computer several times. You will see that the turtle moves a different random number of pixels each time you run the command. Also, the turtle will turn right by a different random number of degrees each time.
REPEAT 100 [FORWARD RANDOM 80 RIGHT RANDOM 90]
More information RANDOM command is at this link.
PICK Command
LOGO has an additional command called PICK that lets us pick a number randomly from a list of numbers.
Syntax: PICK list
Example: The command PICK [1 5 7 2 15] will randomly pick one of the numbers from the list [1 5 7 2 15]. If you run this command again, it will pick a different number from the list [1 5 7 2 15].
Let’s see how we can use the PICK command to randomly select the SIDES parameter in the program example POLYGON :SIDES :LENGTH :COLOR on the Parameters page.
In that example, the main program called the POLYGON procedure with the statement POLYGON 8 40 12. In the modified program, we will replace the SIDES parameter “8” with PICK [6 8 10].
POLYGON PICK[6 8 10] 40 12
When the above program is run, the PICK command will randomly pick a number from the list [6 8 10] and draw a polygon with either 6 sides, or 8 sides, or 10 sides. If you run this program repeatedly, the turtle will draw polygons of different sides (hexagon, octagon, or decagon).
PROGRAM EXAMPLE: PICK COMMAND TO RANDOMLY PICK THE NUMBER OF SIDES FROM A LIST
TO POLYGON :SIDES :LENGTH :COLOR
SETPENCOLOR :COLOR
SETPENSIZE 3
repeat :SIDES[FORWARD :LENGTH RIGHT 360/:SIDES]
HIDETURTLE
End
; MAIN PROGRAM BELOW.
POLYGON PICK[6 8 10] 40 12
Below is the program output. Note that the program picked the SIDES parameter as 6 in this case. If you run the program again, the program may randomly pick a different number from the list [6, 8, 10] and draw a hexagon, or octagon, or decagon.
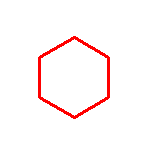
Use PICK Command to Randomly Select Polygon Length
If you decided to pick the length of the polygon randomly from a list (instead of number of sides), you will use the PICK command to select the LENGTH parameter with the command
POLYGON 8 PICK[40 60 80] 12.
In this case, the program will randomly select the length of the sides from the list [40 60 80] and draw an octagon with sides selected randomly from the list [40 60 80].
You can also randomly pick a color of the polygon by using the PICK command for the color parameter. The command
POLYGON 8 40 PICK[4 6 9 14]
will draw an octagon with a length = 40 pixels. The program will randomly select a color from the list [4 6 9 14]. Depending on the number picked from the list, the octagon’s outline color will be different each time you run the program.
From the index table, 4 = red, 6 = yellow, 9 = light brown, 14 = orange. Try it on your computer and see how the polygon outline color changes as you run the program repeatedly.
Miscellaneous Additional Commands
Below are a few more commands that are useful in LOGO programming. These commands include commands to hide the turtle or to make it visible. Other commands discussed in this section enable us to position the turtle at specified coordinates on the screen. The following table lists a few of these commands.
COMMAND | DESCRIPTION | EXAMPLE |
---|---|---|
HIDETURTLE (or HT) | Makes the turtle invisible. To make the turtle visible again, call SHOWTURTLE (or ST). | HIDETURTLE |
SHOWTURTLE (or ST) | Makes the turtle visible. This is the opposite of HIDETURTLE. | SHOWTURTLE |
HOME | Moves the turtle to its HOME position coordinates (0,0). | HOME |
CLEARSCREEN (or CS) | Clears the screen window and send the turtle to its initial position and heading. | CLEARSCREEN |
SETXY x-number y-number | Moves the turtle to (X,Y) coordinates. (X,Y) are the coordinates, where you want the turtle to move to. | SETXY 20 30 Moves the turtle to coordinates (20,30) |
SETPOS position | Moves the turtle to (X,Y) coordinates. The position input is a list of two numbers, the X and Y coordinates. |
Positioning the Turtle
In the examples below, we will show how to use these miscellaneous commands.
HOME Command
If you want to move the turtle to its HOME position, the HOME instruction will move the turtle to the center of the screen and sets the turtle’s position to (0,0).
Instruction format: HOME
SETXY Command
The SETXY instruction is used to move the turtle to an absolute location in its playground. For example, if you want to move the turtle from its HOME position to a different location, you can use the SETXY instruction by specifying the x-coordinate and y-coordinate where you want the turtle to move.
SETXYx-coordinate
y-coordinate
SETPOS Command
SETPOS instruction moves the turtle to an absolute (X,Y) coordinate.
The instruction format is
SETPOS x-coordinate
y-coordinate
The position input is a list of two numbers, the X and Y coordinates.
The SETPOS command can be used to draw a square. See the code below.
SETPOS [0 100]; Move the turtle from HOME position (0,0) to (0,100)
SETPOS [100 100]; Move the turtle to position (100, 100)
SETPOS [100 0]; Move the turtle to position (100,0)
SETPOS [0 0]; Move the turtle to its HOME position (0,0)
Let us now explore how we can use the SETXY command and HIDETURTLE command to Draw A Circle.
SETXY And HIDETURTLE Commands Usage
The program below uses the SETXY and HIDETURTLE (HT) commands. The program has the following steps:
- First set turtle’s pen up using PENUP command the. This needs to be done before you use the SETXY command. If we do not set the pen up, the turtle will draw a line from its HOME position to the turtle’s new (x,y) position as it moves to its new position.
- Next, the command SETXY -250 100 moves the turtle to its new position at coordinates (-250, 100), near the screen’s top left-hand corner.
- The HIDETURTLE command makes the turtle invisible and will not show on the final shape we will draw in step 5.
- Use the command PENDOWN to enable the turtle to draw a trace.
- Finally, the CIRCLE 50 command draws a circle of radius 50 with its center at coordinates (-250, 100).
PROGRAM EXAMPLE: SETXY AND HIDETURTLE COMMANDS USAGE
PENUP ; Step 1)
SETXY -250 100 ; Step 2)
HIDETURTLE ; Step 3)
PENDOWN ; Step 4)
CIRCLE 50 ; Step 5)
The following is the program’s output.
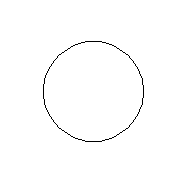
Copyright © 2019 – 2024 softwareprogramming4kids.com
All Rights Reserved